C++ Program Structure
Let us look at a
simple code that would print the words Hello World.
#include <iostream>
using namespace std;
using namespace std;
// main() is where program execution begins.
int main()
{
cout << "Hello World"; // prints Hello World
return 0;
}
{
cout << "Hello World"; // prints Hello World
return 0;
}
Let us look various
parts of the above program:
- The C++ language defines several headers, which contain information that is either necessary or useful to your program. For this program, the header <iostream> is needed.
- The line using namespace std; tells the compiler to use the std namespace. Namespaces are a relatively recent addition to C++.
- The next line // main() is where program execution begins. is a single-line comment available in C++. Single-line comments begin with // and stop at the end of the line.
- The line int main() is the main function where program execution begins.
- The next line cout << "This is my first C++ program."; causes the message "This is my first C++ program" to be displayed on the screen.
- The next line return 0; terminates main( )function and causes it to return the value 0 to the calling process.
Data Types
The following table
shows the variable type, how much memory it takes to store the value in memory,
and what is maximum and minimum value which can be stored in such type of
variables.
Type
|
Typical Bit Width
|
Typical Range
|
char
|
1byte
|
-127
to 127 or 0 to 255
|
unsigned
char
|
1byte
|
0 to
255
|
signed
char
|
1byte
|
-127
to 127
|
int
|
4bytes
|
-2147483648
to 2147483647
|
unsigned
int
|
4bytes
|
0 to
4294967295
|
signed
int
|
4bytes
|
-2147483648
to 2147483647
|
short
int
|
2bytes
|
-32768
to 32767
|
unsigned
short int
|
Range
|
0 to
65,535
|
signed
short int
|
Range
|
-32768
to 32767
|
long
int
|
4bytes
|
-2,147,483,648
to 2,147,483,647
|
signed
long int
|
4bytes
|
same
as long int
|
unsigned
long int
|
4bytes
|
0 to
4,294,967,295
|
float
|
4bytes
|
+/-
3.4e +/- 38 (~7 digits)
|
double
|
8bytes
|
+/-
1.7e +/- 308 (~15 digits)
|
long
double
|
8bytes
|
+/-
1.7e +/- 308 (~15 digits)
|
wchar_t
|
2 or
4 bytes
|
1
wide character
|
C++ Keywords
The following list
shows the reserved words in C++. These reserved words may not be used as
constant or variable or any other identifier names.
asm
|
else
|
new
|
this
|
auto
|
enum
|
operator
|
throw
|
bool
|
explicit
|
private
|
true
|
break
|
export
|
protected
|
try
|
case
|
extern
|
public
|
typedef
|
catch
|
false
|
register
|
typeid
|
char
|
float
|
reinterpret_cast
|
typename
|
class
|
for
|
return
|
union
|
const
|
friend
|
short
|
unsigned
|
const_cast
|
goto
|
signed
|
using
|
continue
|
if
|
sizeof
|
virtual
|
default
|
inline
|
static
|
void
|
delete
|
int
|
static_cast
|
volatile
|
do
|
long
|
struct
|
wchar_t
|
double
|
mutable
|
switch
|
while
|
dynamic_cast
|
namespace
|
template
|
Variable
A
variable provides us with named storage that our programs can manipulate.
DataType variableName1,
variableName2,…variableNameN;
int
i; // declared but not
initialised
char c;
int i, j, k; // Multiple declaration
char c;
int i, j, k; // Multiple declaration
int
i=10; //initialization and
declaration in same step
int i=10, j=11;
int i=10, j=11;
If
a variable is declared and not initialized by default it will hold a garbage
value. Also, if a variable is once declared and if try to declare it again, we
will get a compile time error.
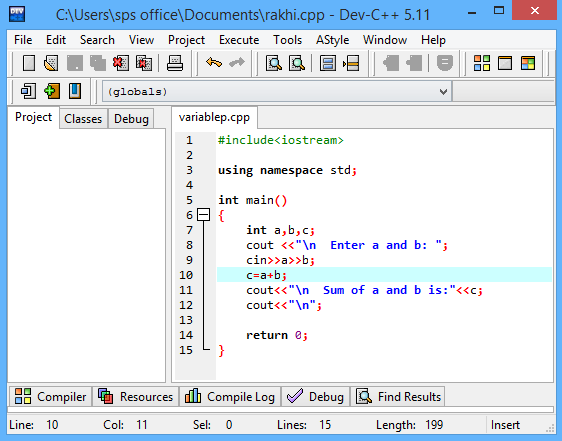
Loop
A
loop statement allows us to execute a statement or group of statements multiple
times and following is the general from of a loop statement in most of the
programming languages.
There are 3 type of loops in C++ language
Variable initialization.( e.g int x=0; )There are 3 type of loops in C++ language
while
loop
while loop can be
address as an entry
control loop. It is completed in 3
steps.
condition( e.g while( x<=10) )
Variable increment or decrement ( x++ or x-- or x=x+2 )
Syntax
variable initialization ;
while (condition)
{
statements ;
variable increment or decrement ;
For
loop
For loop is used
to execute a set of statement repeatedly until a particular condition is
satisfied. we can say it an open ended loop. General
format is,
for(initialization; condition ;
increment/decrement)
{
statement-block;
}
Nested
for loop
We
can also have nested for loop, i.e one for loop inside another for loop. Basic
syntax is,
for(initialization; condition;
increment/decrement)
{
for(initialization; condition;
increment/decrement)
{
statement ;
}
do while loop
In
some situations it is necessary to execute body of the loop before testing the
condition. Such situations can be handled with the help of do-while loop. do statement
evaluates the body of the loop first and at the end, the condition is checked
using while statement.
General format of do-while loop is,
do
{
....
.....
}
while(condition)
The Infinite Loop
A
loop becomes infinite loop if a condition never becomes false.
C++
programmers more commonly use the for(;;) construct to signify an infinite
loop.
#include
<iostream>
using namespace std;
int main ()
{
using namespace std;
int main ()
{
for(
; ; )
{
printf("This loop will run forever.\n");
}
{
printf("This loop will run forever.\n");
}
return
0;
}
Decision making in C++
Decision making is about deciding the order of execution of statements based on certain conditions or repeat a group of statements until certain specified conditions are met. C++ handles decision-making by supporting the following statements,
Simple if statement
The general form of a simple if statement is,
}
Decision making is about deciding the order of execution of statements based on certain conditions or repeat a group of statements until certain specified conditions are met. C++ handles decision-making by supporting the following statements,
Simple if statement
The general form of a simple if statement is,
if( expression
)
{
statement-inside;
}
statement-outside;
{
statement-inside;
}
statement-outside;
If
the expression is
true, then 'statement-inside' it will be executed, otherwise 'statement-inside'
is skipped and only 'statement-outside' is executed.
if...else statement
The general form of a simple if...else statement is,
if...else statement
The general form of a simple if...else statement is,
if( expression
)
{
statement-block1;
}
else
{
statement-block2;
}
If the 'expression' is true, the 'statement-block1' is executed, else 'statement-block1' is skipped and 'statement-block2' is executed.
Nested if....else statement
The general form of a nested if...else statement is,
{
statement-block1;
}
else
{
statement-block2;
}
If the 'expression' is true, the 'statement-block1' is executed, else 'statement-block1' is skipped and 'statement-block2' is executed.
Nested if....else statement
The general form of a nested if...else statement is,
if( expression
)
{
if( expression1 )
{
statement-block1;
}
else
{
statement-block 2;
}
}
else
{
statement-block 3;
}
if 'expression' is false the 'statement-block3' will be executed, otherwise it continues to perform the test for 'expression 1' . If the 'expression 1' is true the 'statement-block1' is executed otherwise 'statement-block2' is executed.
else-if ladder
The general form of else-if ladder is,
{
if( expression1 )
{
statement-block1;
}
else
{
statement-block 2;
}
}
else
{
statement-block 3;
}
if 'expression' is false the 'statement-block3' will be executed, otherwise it continues to perform the test for 'expression 1' . If the 'expression 1' is true the 'statement-block1' is executed otherwise 'statement-block2' is executed.
else-if ladder
The general form of else-if ladder is,
if(expression
1)
{
statement-block1;
}
else if(expression 2)
{
statement-block2;
}
else if(expression 3 )
{
statement-block3;
}
else
{
statement-block1;
}
else if(expression 2)
{
statement-block2;
}
else if(expression 3 )
{
statement-block3;
}
else
default-statement;
The expression is tested from the top(of the ladder) downwards. As soon as the true condition is found, the statement associated with it is executed.
Functions in C++
Functions are used to provide modularity to a program. Creating an application using function makes it easier to understand, edit, check errors etc.
Syntax of Function
return-type function-name (parameters)
{
// function-body
}
The expression is tested from the top(of the ladder) downwards. As soon as the true condition is found, the statement associated with it is executed.
Functions in C++
Functions are used to provide modularity to a program. Creating an application using function makes it easier to understand, edit, check errors etc.
Syntax of Function
return-type function-name (parameters)
{
// function-body
}
- return-type : suggests what the function will return. It can be int, char, some pointer or even a class object. There can be functions which does not return anything, they are mentioned with void.
- Function Name : is the name of the function, using the function name it is called.
- Parameters : are variables to hold values of arguments passed while function is called. A function may or may not contain parameter list.
- Function body : is he part where the code statements are written.
Call by Value
In
this calling technique we pass the values of arguments which are stored or
copied into the formal parameters of functions. Hence, the original values are
unchanged only the parameters inside function changes.
Call by Reference
In this we pass the address of the variable as arguments. In this case the formal parameter can be taken as a reference or a pointer, in both the case they will change the values of the original variable.
Array
C++ provides a data structure, the array, which stores a fixed-size sequential collection of elements of the same type.
An
array is a sequence of variable that can store value of one particular data
type.
type array_name[size];
int age[5];
This array can hold 5 integer elements.
C++ Multidimensional Arrays
C++ allows programmer to create array of an array known as multidimensional arrays. Consider this example:
int x[3][4];
Here, x is a two dimensional array. This array can hold 12 elements. You can think this array as table with 3 row and each row has 4 column.
type array_name[size];
int age[5];
This array can hold 5 integer elements.
C++ Multidimensional Arrays
C++ allows programmer to create array of an array known as multidimensional arrays. Consider this example:
int x[3][4];
Here, x is a two dimensional array. This array can hold 12 elements. You can think this array as table with 3 row and each row has 4 column.
String in C++
String
is a sequence of characters.
The
standard C++ library provides a string class type that supports wide range of functions that
manipulate null-terminated strings.
strcpy(s1, s2)
Copies
string s2 into string s1.
strcat(s1, s2)
Concatenates
string s2 onto the end of string s1.
strlen(s1)
Returns
the length of string s1.
strcmp(s1, s2)
Returns
0 if s1 and s2 are the same; less than 0 if s1<s2; greater than 0 if
s1>s2.
strchr(s1, ch)
Returns
a pointer to the first occurrence of character ch in string s1.
strstr(s1, s2)
Returns
a pointer to the first occurrence of string s2 in string s1.
C++ Tutorial
Reviewed by Unknown
on
04:19:00
Rating:
No comments: