Creating the Program
A Perl
program consists of an ordinary text file containing a series of Perl
statements. Statements are written in what looks like an amalgam of C, UNIX
shell script, and English.Perl code can be quite free-flowing. The broad
syntactic rules governing where a statement starts and ends are:
- Leading spaces on a line are ignored. You can start a Perl statement anywhere you want: at the beginning of the line, indented for clarity (recommended) or even right-justified (definitely frowned on because the code would be difficult to understand) if you like.
- Statements are terminated with a semicolon.
- Spaces, tabs, and blank lines outside of strings are irrelevant-one space is as good as a hundred. That means you can split statements over several lines for clarity. A string is basically a series of characters enclosed in quotes.
- Anything after a hash sign (#) is ignored except in strings. Use this fact to pepper your code with useful comments.
Comments
In most programming languages you can add comments to the code which are ignored by the compiler/interpreter. This is used to increase the readability of the code. • In Perl, everything between a # and the end of a line is treated as a comment.
print "Hello
World!"; # prints Hello World! to the screen.
Datatypes
Perl has three basic data types − scalars, arrays of scalars, and hashes of scalars, also known as associative arrays. Here is a little detail about these data types.
S.N.
|
Types and Description
|
1
|
Scalar −
Scalars
are simple variables. They are preceded by a dollar sign ($). A scalar is
either a number, a string, or a reference. A reference is actually an address
of a variable, which we will see in the upcoming chapters.
|
2
|
Arrays −
Arrays
are ordered lists of scalars that you access with a numeric index which
starts with 0. They are preceded by an "at" sign (@).
|
3
|
Hashes −
Hashes
are unordered sets of key/value pairs that you access using the keys as
subscripts. They are preceded by a percent sign (%).
|
Variable
Creating
Variables
Perl variables do not
have to be explicitly declared to reserve memory space. The declaration happens
automatically when you assign a value to a variable. The equal sign (=) is used
to assign values to variables.
$age = 25; # An integer assignment
$name = "John Paul"; # A string
$salary = 1445.50; # A floating point
$name = "John Paul"; # A string
$salary = 1445.50; # A floating point
Array
An array, in Perl, is an ordered list of scalar data.
Each
element of an array is an separate scalar variable with a independent scalar
value -- unlike PASCAL or C.
@ages = (25, 30,
40);
@names = ("John Paul", "Lisa", "Kumar");
@names = ("John Paul", "Lisa", "Kumar");
conditional statements
If
if
(expression)
{ true_statement_1;
.....
true_statement_n;
}
{ true_statement_1;
.....
true_statement_n;
}
If..else
if
(expression)
{ true_statement_1;
.....
true_statement_n;
}
else
{ false_statement_1;
.....
false_statement_n;
}
{ true_statement_1;
.....
true_statement_n;
}
else
{ false_statement_1;
.....
false_statement_n;
}
If..elseif..else
if
(expression)
{ true_statement_1;
.....
true_statement_n;
}
else
{ false_statement_1;
.....
false_statement_n;
}
{ true_statement_1;
.....
true_statement_n;
}
else
{ false_statement_1;
.....
false_statement_n;
}
Loop
For loop
A for loop counts
through a range of numbers, running a block of code each time it iterates
through the loop.
for (
initialise_expr; test_expr; increment_expr )
{
statement(s);
}
{
statement(s);
}
while loop
While loops
continually iterate as long as the conditional statement remains true.
while
(expression)
{ # while expression is true execute this block
{ # while expression is true execute this block
statement(s);
}
}
Until loop
Repeats a statement or group of statements until a given condition becomes true. It tests the condition before executing the loop body.
Do..while loop
Like a while
statement, except that it tests the condition at the end of the loop body
Function
A function
definition is very simple. It consists of:
sub
functionName {
}
perl - hashes
Hashes are
complex list data, like arrays except they link a key to a value. To define a
hash, we use the percent (%) symbol before the name.
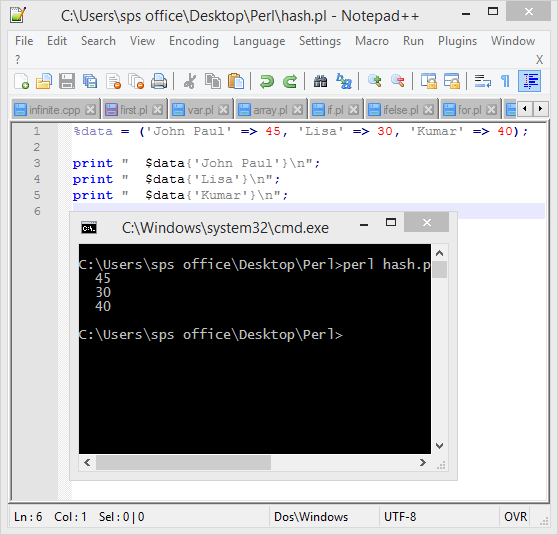
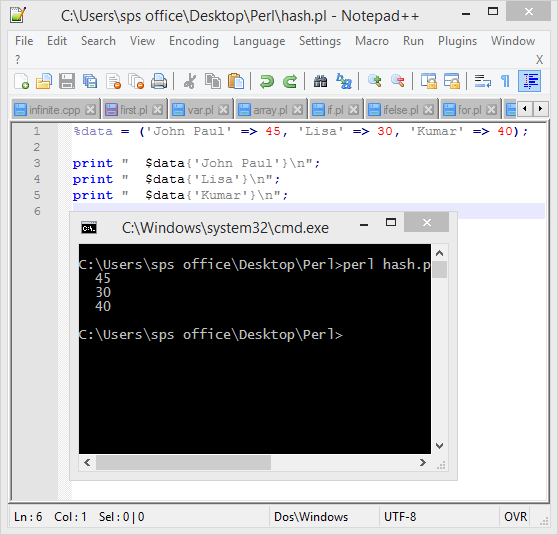
String Functions
Perl
provides a very useful set of string handling functions:
lc, uc, length
There are a number of simple functions such as lc and uc to return the lower case and upper case versions of the original string respectively. Then there islength to return the number of characters in the given string.
Index
This function will get two strings and return the location of the second string within the first string.
substr
substr will give
you the substring
at a given locations. Normally substr gets
3 parameters. The first one is the string. The second is a 0-based location,
also called the offset, and the third is the length of the substring we would
like to get.
Perl Tutorial
Reviewed by Unknown
on
05:26:00
Rating:

No comments: